Your costs = usage. Period.
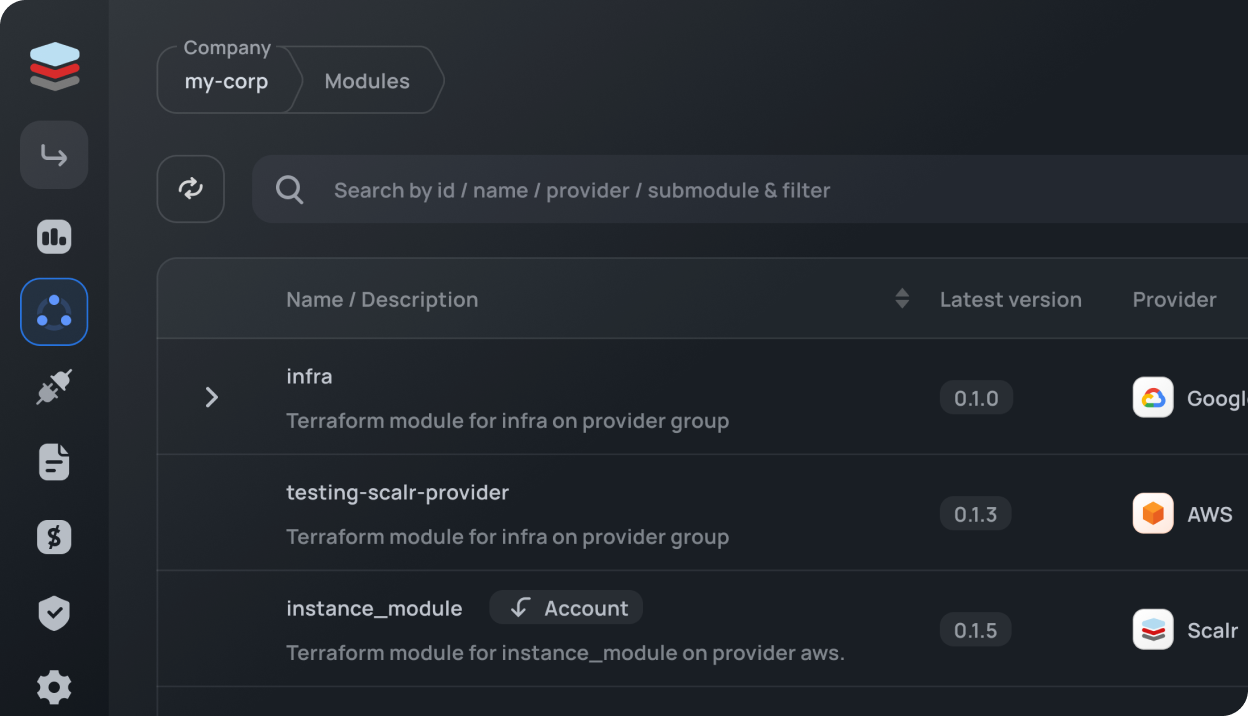
There are a couple of core components in any infrastructure as code pipelines: An IaC platform, such as Terraform or OpenTofu, and a version control system (VCS) such as Bitbucket. Terraform, a widely adopted IaC tool, allows users to define and provision infrastructure using a declarative configuration language. Integrating Terraform with Bitbucket adds an extra layer of control and collaboration. When talking about Bitbucket with Terraform, many think about pull request automation, which we will touch on in the second half of this blog, but we’ll also focus on how to use Terraform to manage your Bitbucket configuration as well as the integration Scalr has with Bitbucket
Terraform uses the concept of providers to interact with endpoint APIs to create resources in the provider. The Bitbucket Terraform provider serves as a bridge between your infrastructure code and Bitbucket Cloud or Bitbucket Datacenter. It allows you to define and manage Bitbucket resources – such as repositories and projects – directly within your Terraform modules and code. This integration ensures that your repository configurations are codified, version-controlled, and can be easily reproduced across different environments.
An important aspect of managing Bitbucket with Terraform is to select the appropriate Terraform provider to use. While many other tools, like Okta and Gitlab, have officially supported providers, Bitbucket is community driven so it’s important to select the provider that is maintained and used the most. When searching in the standard Terraform registry, it was hard to figure out which provider to go with as there aren’t really many insights provided.
Instead, I used library.tf, a registry maintained by Scalr, which gave me the insights I needed to make the decision on which provider to use:
As seen above, there is only one provider that has been updated in the last year and has frequent commits, when clicking on the provider I can also see the number of contributors, open issues, and more to help me make my decision on using this Bitbucket provider.
Before getting into the common use cases of using the Bitbucket Terraform provider, ensure that you have the following prerequisites in place:
In the following steps, we’ll provide you with the methods to authenticate to the provider and then walk through some basic examples of using it.
Start by configuring the Bitbucket Terraform provider in your Terraform code. Open your Terraform configuration file (commonly named main.tf) and add the following block:
terraform {
required_providers {
bitbucket = {
source = "DrFaust92/bitbucket"
version = "2.40.1"
}
}
}
provider "bitbucket" {
username = "your-username"
password = "your-password"
}
Replace "your-username” with your Bitbucket username and "your-password” with the password you use for Bitbucket. The latest version of the Bitbucket Terraform provider documentation can be found here.
Now, let's create a Bitbucket repository using the Bitbucket Terraform resource. This can all be viewed in detail in the Terraform registry here . Add the following code to your configuration:
resource "bitbucket_repository" "example_repo" {
owner = "user or team name"
name = "repo-name"
is_private = true
}
This Terraform code defines a resource "example_repo" that is set to be private.
In this example we’ll manage the groups in Bitbucket and what permissions they have. In this case, we’ll also use a datasource to pull the workspace ID:
data "bitbucket_workspace" "example" {
workspace = "example"
}
resource "bitbucket_group" "example_group" {
workspace = data.bitbucket_workspace.example.id
name = "example group"
permission = "read"
}
This Terraform code defines a resource "example_group" and sets the groups permissions to read.
Once you've defined your Bitbucket resources through the Bitbucket Terraform provider, navigate to the directory containing your Terraform configuration file and run the following commands:
terraform init
terraform plan
terraform apply
If you are using OpenTofu, use the tofu commands instead:
tofu init
tofu plan
tofu apply
Terraform will initialize the Bitbucket provider and apply the changes to your Bitbucket account. Upon a successful Terraform run, the state file will be created.
Utilize Terraform variables to make your configurations more dynamic. Instead of hardcoding values, use variables to create reusable and flexible scripts.
variable "username" {
description = "The username you authenticate to Bitbucket with"
}
variable "password" {
description = "The password you authenticate to Bitbucket with"
}
provider "bitbucket" {
org_name = var.username
api_token = "var.password"
}
Consider using remote state management to store your Terraform state files securely. Services like Scalr or AWS S3 can be configured as remote backends to store state files. Here is an example of connecting to Scalr:
terraform {
backend "remote" {
hostname = "<account-name>.scalr.io"
organization = "<scalr-environment-name>"
workspaces {
name = "<workspace-name>"
}
}
}
To improve your Terraform code, we encourage you to review the option of using Bitbucket data sources in the code to be able to pull information from other resources or workspaces into the run.
This guide covers the basic steps to configure the Bitbucket Terraform provider, create repositories, and groups, and introduce best practices for advanced usage. As you explore further, consider exploring additional Bitbucket resources supported by the Bitbucket provider, such as branches, deploy keys, and projects. The library.tf documentation for the Bitbucket Terraform provider is a valuable resource for in-depth information and examples.
By integrating Bitbucket into your Terraform workflows, you're not just managing infrastructure – you're managing your VCS provider with the efficiency and scalability that infrastructure as code brings.
Now we’re going to switch gears a little and talk about how Bitbucket can be used as a vital integration in your overall Terraform pipeline and how it integrates with Scalr.
When talking about Terraform and Bitbucket, the number one best practice is to make sure your Terraform files are stored in a VCS repository, like Bitbucket. By storing the Terraform configuration in a Bitbucket repository, you enable the following for your infrastructure teams:
If you are looking for a TACO, such as Scalr or Terraform Cloud, to help scale your Terraform operations, the integration with Bitbucket is a key feature. By integrating these tools with Bitbucket, you are not only able to pull in the Terraform code but also enable the following features:
The TACO products have a full feature set dedicated to Terraform, including the ability to store Terraform state files. See more here.